3 Ways to Implement Datepicker Input in Rails 6We look at ways to implement a date picker input in the newer version of Rails
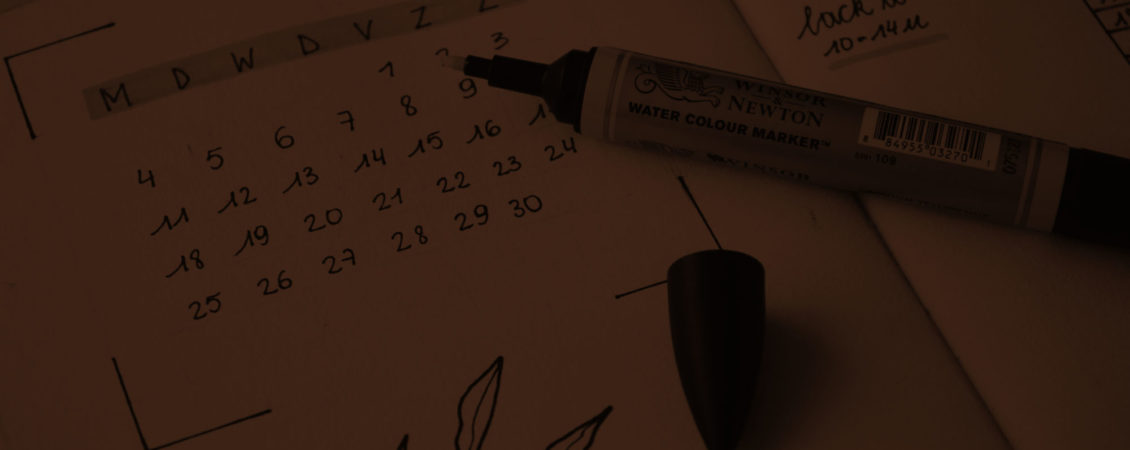
In this article we will look at 3 ways to implement a datepicker input in Rails 6.0. We will first look at the default date_field
and then look at two gems to implement this. You can view the demo of this at https://datepicker-rails6.herokuapp.com/.
1. Using the date_field
form helper
When using the date_field
form helper is the easiest way to implement the date picker input in Rails. This renders the browser specific datepicker widget, corresponding to the <input type='date'>
. The datepicker shown is visually different in different browsers. You can read more about this in the Mozilla MDN docs for datepicker.
2. Bootstrap Datepicker (bootstrap-datepicker-rails
gem)
Another way to add a date picker input is using the gem bootstrap-datepicker-rails
. This is an asset pipeline wrapper over a date picker build with Bootstrap 4 – https://github.com/uxsolutions/bootstrap-datepicker.
Lets say you have a text field release_date
in a Rails 6 form. Add that as a text_field
.
<%= form_with do |f|%>
...
<%= f.text_field :release_date, class: 'form-control %>
...
<%end%>
Let us start by adding gem to our application.
# File: Gemfile
gem 'bootstrap-datepicker-rails'
This gem uses the traditional asset pipeline. So the css and js assets has to be added to app/assets/stylesheets/application.css
.
/* File: app/assets/stylesheets/application.css */
/*
*= require bootstrap-datepicker
*= require_self
*/
And to app/assets/javascripts/application.js
. Also add jQuery
, bootstrap and popper javascripts to application.js.
# File: app/assets/javascripts/application.js
//= require jquery-3.2.1.slim.min
//= require popper.min
//= require bootstrap.min
//= require bootstrap-datepicker/core
//= require bootstrap-datepicker/locales/bootstrap-datepicker.en-GB.js
By default, app/assets/javascripts/application.js
is not included in the asset pipeline. Add it in the rails initializer – initializers/assets.rb
.
# File: config/initializers/assets.rb
# ...
Rails.application.config.assets.precompile += %w( application.js )
To have application.js
of the asset pipeline in the application layout add it using javascript_include_tag
.
<%= javascript_include_tag 'application', 'data-turbolinks-track': 'reload' %>
Now for the widget to appear in the text field, add the class ‘datepicker’ to the text_field
.
<%= f.text_field :release_date, class: 'form-control datepicker' %>
In app/assets/javascripts/application.js
file initialize the datepicker using javascript.
// File: app/assets/javascripts/application.js
// ...
// ...
$(document).ready(function(){
$('.datepicker').datepicker({
format: 'dd/mm/yyyy'
});
});
You can also use data-*
attributes to initialize the datepicker.
Refer to the source code in this commit for the implementation.
Additional configuration options:
You can use the following configuration options, passed to the datepicker()
js initializer.
format: 'dd/mm/yyyy'
for changing the date format of the date pickerstartDate: '0d'
to disable all past dates.endDate: '+1y'
to enable only dates till one year rom now.language: 'de'
to change the language to German.weekStart: 1
to change the start of week to Monday. 0 (Sunday) to 6 (Saturday)
3. Bootstrap 4 Datetime Picker (bs4_datetime_picker
gem)
Compared to the other datepickers mentioned above, this is a date-time picker. You have the choice to pick a time as well. This is based on Tempus Dominus Bootstrap4 Datetime Picker js. The gem is an asset pipeline wrap, and is available at https://github.com/rdavid369/bs4-datetime-picker.
This too requires changes to the Gemfile, and application.css
and application.js
files; and is dependent on jQuery and Bootstrap 4. (As mentioned above application.js has to be created and has to be added to the asset pipeline, by modifying the initializers/assets.rb
file.)
# File: Gemfile
# ...
gem 'bs4_datetime_picker'
gem 'font-awesome-rails'
The following changes are to be made in application.css
. Some of the icons in this date-time picker depends on font-awesome. So that has to be included to the css.
/* File: app/assets/stylesheets/application.css */
/*
*= require_tree .
*= require font-awesome
*= require bs4-datetime-picker
*= require_self
*/
It depends on momentJS and it has to be included to application.js
as well.
// File: app/assets/javascripts/application.js
//= require jquery-3.2.1.slim.min
//= require popper.min
//= require bootstrap.min
//= require moment
//= require bs4-datetime-picker
The date field in the form has to be customised as follows:
<div class="form-group">
<%= f.label :release_date %>
<div class="input-group date" id="datetimepicker1" data-target-input="nearest">
<%= f.text_field :release_date, class: 'form-control datetimepicker-input', 'data-target': "#datetimepicker1" %>
<div class="input-group-append" data-target="#datetimepicker1" data-toggle="datetimepicker">
<div class="input-group-text"><i class='fa fa-calendar'></i></div>
</div>
</div>
<small class="form-text text-muted">Date when the product will be released.</small>
</div>
And finally initialize the datetime picker in application.js
as follows:
// File: app/assets/javascripts/application.js
// ...
// ...
$(document).ready(function(){
$('#datetimepicker').datetimepicker();
});
This brings up the date-time picker widget on click.
The date-time has to be parsed in the controller appropriately, before saving to the database.
Refer to the source code in this commit for the implementation.
Conclusion
We saw different ways to implement a date picker in Rails 6. The full source code for the sample code is available in the Github Repository.